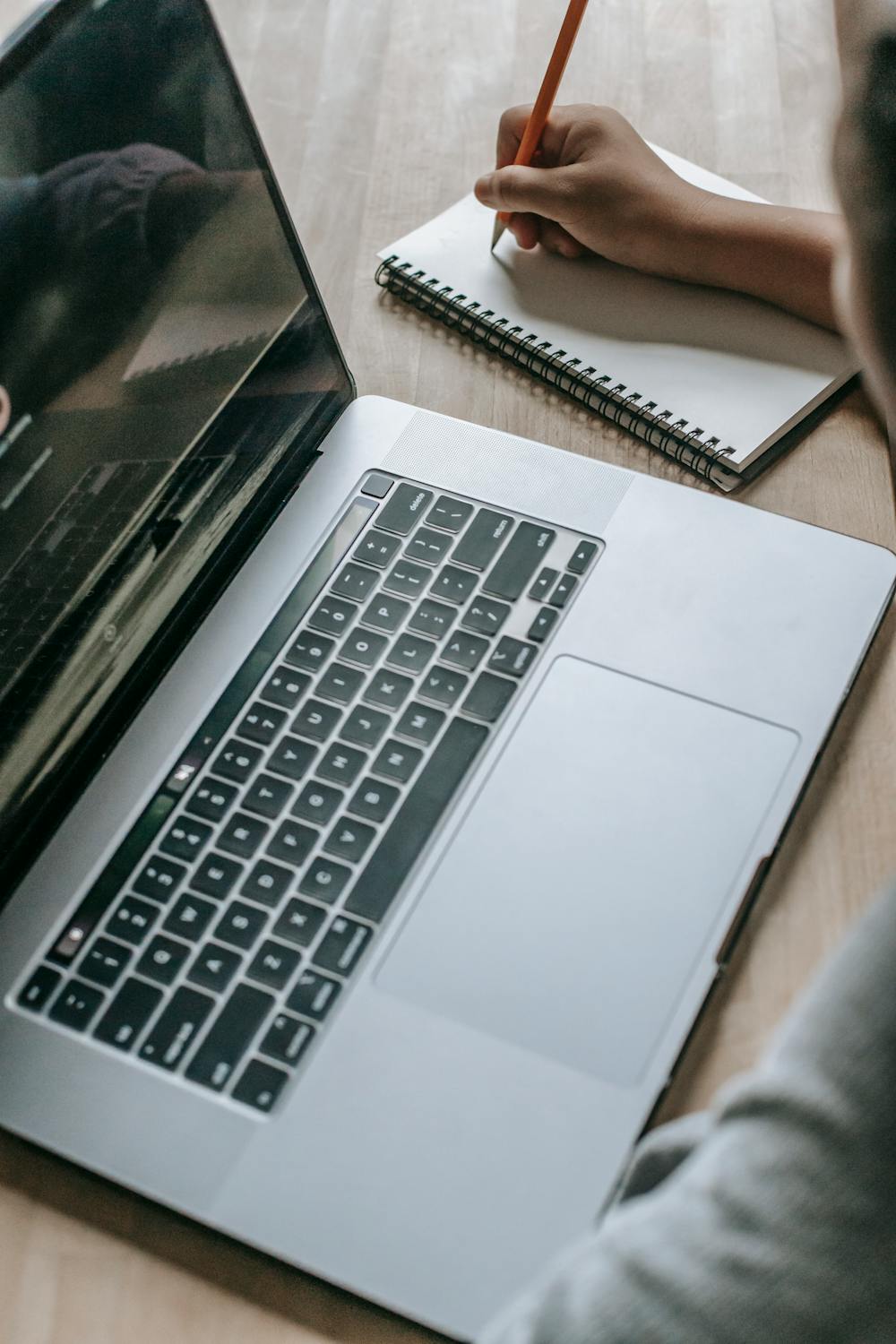
Automating Accessibility Compliance with Cypress
Catch accessibility issues early in your development workflow by integrating automated checks into your end-to-end tests. Learn how Cypress and the cypress-axe plugin can help you enforce WCAG standards continuously, from local development through CI pipelines.
Automating Accessibility Compliance with Cypress
Catch accessibility issues early in your development workflow by integrating automated checks into your end-to-end tests. Learn how Cypress and the cypress-axe plugin enforce WCAG standards continuously, from local development through CI.
Introduction
Ensuring your web application meets accessibility standards isn’t just good practice—it’s a legal requirement and a moral imperative. Manual audits catch only a fraction of potential issues. Automating accessibility checks as part of your test suite helps you catch regressions immediately, reduce remediation costs, and deliver a more inclusive user experience.
Why Automate Accessibility Testing?
- Early Detection: Identify color-contrast failures, missing ARIA attributes, and keyboard navigation gaps before they hit production.
- Continuous Enforcement: Gate pull requests so every merge maintains compliance.
- Scalability: Run the same tests against multiple pages, components, and viewports without extra manual effort.
Setup: Cypress + cypress-axe
-
Install dependencies
npm install --save-dev cypress cypress-axe axe-core # or yarn add --dev cypress cypress-axe axe-core
-
Register in support file
In cypress/support/e2e.js:
import 'cypress-axe'; beforeEach(() => { cy.injectAxe(); });
Writing Your First Accessibility Tests
// cypress/e2e/accessibility.spec.js
describe('Accessibility Compliance', () => {
it('Home page has no detectable a11y violations', () => {
cy.visit('/');
cy.checkA11y();
});
it('Component Gallery meets WCAG 2.1 AA', () => {
cy.visit('/components');
cy.get('.component-card').each(($el) => {
cy.wrap($el).checkA11y(null, {
runOnly: {
type: 'tag',
values: ['wcag2aa']
}
});
});
});
});
- cy.injectAxe() initializes axe-core.
- cy.checkA11y() runs the audit.
- Use runOnly to narrow scope to specific WCAG levels.
Customizing Rules & Reporting
Suppress known issues or focus on specific rules:
cy.checkA11y(
null,
{ rules: { 'color-contrast': { enabled: false } } },
(violations) => {
violations.forEach(({ id, description, nodes }) => {
console.log(`${id}: ${description}`, nodes);
});
}
);
Export violations to JSON or integrate with custom reporters for deeper analysis.
Integrating into CI
Add an npm script for headless runs:
"scripts": {
"test:e2e": "cypress run"
}
GitHub Actions example:
jobs:
e2e:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- uses: cypress-io/github-action@v5
with:
command: npm run test:e2e
Failures in accessibility tests will block merges, preventing regressions.
Best Practices
- Baseline Audit: Run a full manual audit with browser devtools at project kickoff.
- Component-Level Tests: Isolate complex widgets to cover ARIA roles and keyboard behavior.
- Visual Testing: Combine axe checks with visual diff tools to catch missing focus indicators.
- Team Training: Share audit reports and pair-program remediation to build accessibility awareness.
Conclusion
Embedding automated accessibility checks with Cypress and cypress-axe transforms your workflow from reactive fixes to proactive quality assurance. Start with critical pages, integrate into CI, then expand coverage as confidence grows—each deployment becomes an opportunity to strengthen usability and inclusivity.